Write a C++ program to print the same numbers on all sides of a square pattern using for loop.
#include<iostream> using namespace std; int main() { int i, j, k, min, rows; cout << "Enter Sqaure Number Rows = "; cin >> rows; cout << "Print Same Number in All Sides of a Square Pattern\n"; for(i = 1; i <= rows; i++) { for(j = 1; j <= rows; j++) { min = i < j? i: j; cout << rows - min + 1 << " "; } for(k = rows - 1; k >= 1; k--) { min = i < k? i:k; cout << rows - min + 1 << " "; } cout << "\n"; } for(i = rows - 1; i >= 1; i--) { for(j = 1; j <= rows; j++) { min = i < j? i:j; cout << rows - min + 1 << " "; } for(k = rows - 1; k >= 1; k--) { min = i < k? i:k; cout << rows - min + 1 << " "; } cout << "\n"; } return 0; }
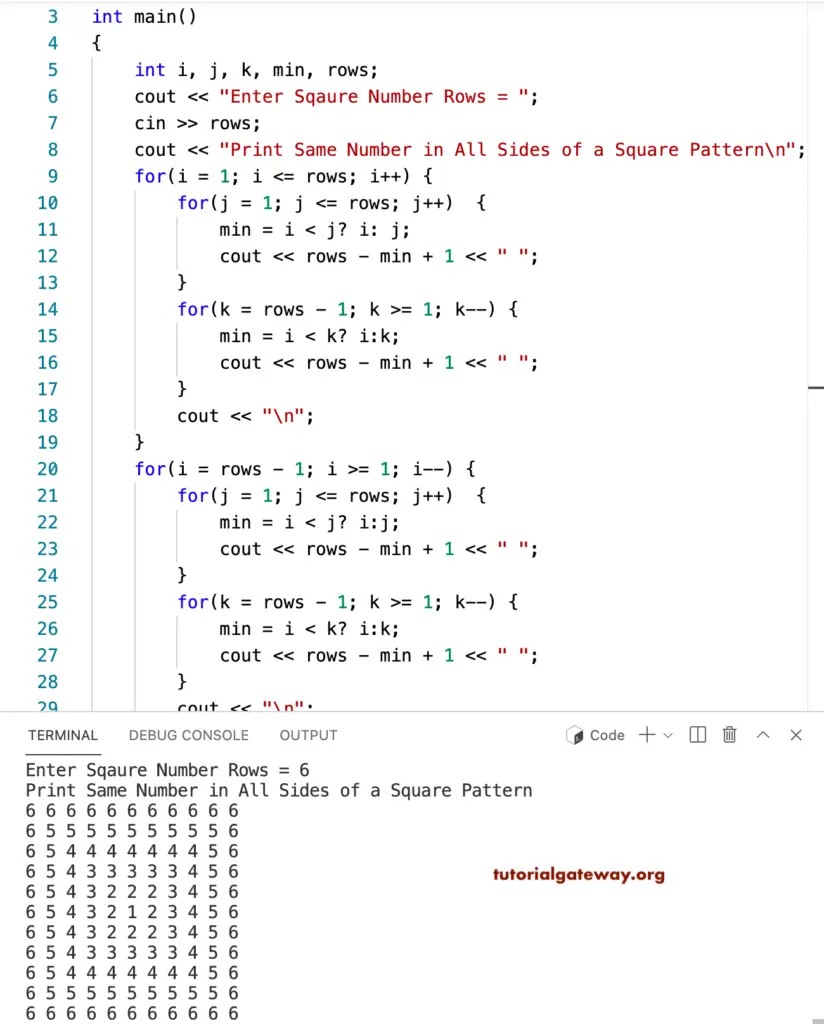
This C++ example prints the square pattern where all sides have the same numbers using a while loop.
#include<iostream> using namespace std; int main() { int i = 1, j, k, min, rows; cout << "Enter Sqaure Number Rows = "; cin >> rows; cout << "Print Same Number in All Sides of a Square Pattern\n"; while( i <= rows) { j = 1; while( j <= rows) { min = i < j? i: j; cout << rows - min + 1 << " "; j++; } k = rows - 1; while( k >= 1) { min = i < k? i:k; cout << rows - min + 1 << " "; k--; } cout << "\n"; i++; } i = rows - 1; while( i >= 1) { j = 1; while( j <= rows) { min = i < j? i: j; cout << rows - min + 1 << " "; j++; } k = rows - 1; while( k >= 1) { min = i < k? i:k; cout << rows - min + 1 << " "; k--; } cout << "\n"; i--; } return 0; }
Enter Sqaure Number Rows = 9
Print Same Number in All Sides of a Square Pattern
9 9 9 9 9 9 9 9 9 9 9 9 9 9 9 9 9
9 8 8 8 8 8 8 8 8 8 8 8 8 8 8 8 9
9 8 7 7 7 7 7 7 7 7 7 7 7 7 7 8 9
9 8 7 6 6 6 6 6 6 6 6 6 6 6 7 8 9
9 8 7 6 5 5 5 5 5 5 5 5 5 6 7 8 9
9 8 7 6 5 4 4 4 4 4 4 4 5 6 7 8 9
9 8 7 6 5 4 3 3 3 3 3 4 5 6 7 8 9
9 8 7 6 5 4 3 2 2 2 3 4 5 6 7 8 9
9 8 7 6 5 4 3 2 1 2 3 4 5 6 7 8 9
9 8 7 6 5 4 3 2 2 2 3 4 5 6 7 8 9
9 8 7 6 5 4 3 3 3 3 3 4 5 6 7 8 9
9 8 7 6 5 4 4 4 4 4 4 4 5 6 7 8 9
9 8 7 6 5 5 5 5 5 5 5 5 5 6 7 8 9
9 8 7 6 6 6 6 6 6 6 6 6 6 6 7 8 9
9 8 7 7 7 7 7 7 7 7 7 7 7 7 7 8 9
9 8 8 8 8 8 8 8 8 8 8 8 8 8 8 8 9
9 9 9 9 9 9 9 9 9 9 9 9 9 9 9 9 9