Write a C++ program to print right triangle of consecutive row alphabets pattern using for loop.
#include<iostream> using namespace std; int main() { int rows, val; cout << "Enter Right Triangle of Consecutive Row Alphabets Rows = "; cin >> rows; cout << "Right Triangle of Consecutive Row Alphabets Pattern\n"; int alphabet = 64; for (int i = 1; i <= rows; i++) { val = i; for (int j = 1; j <= i; j++) { cout << char(alphabet + val) << " "; val = val + rows - j; } cout << "\n"; } }
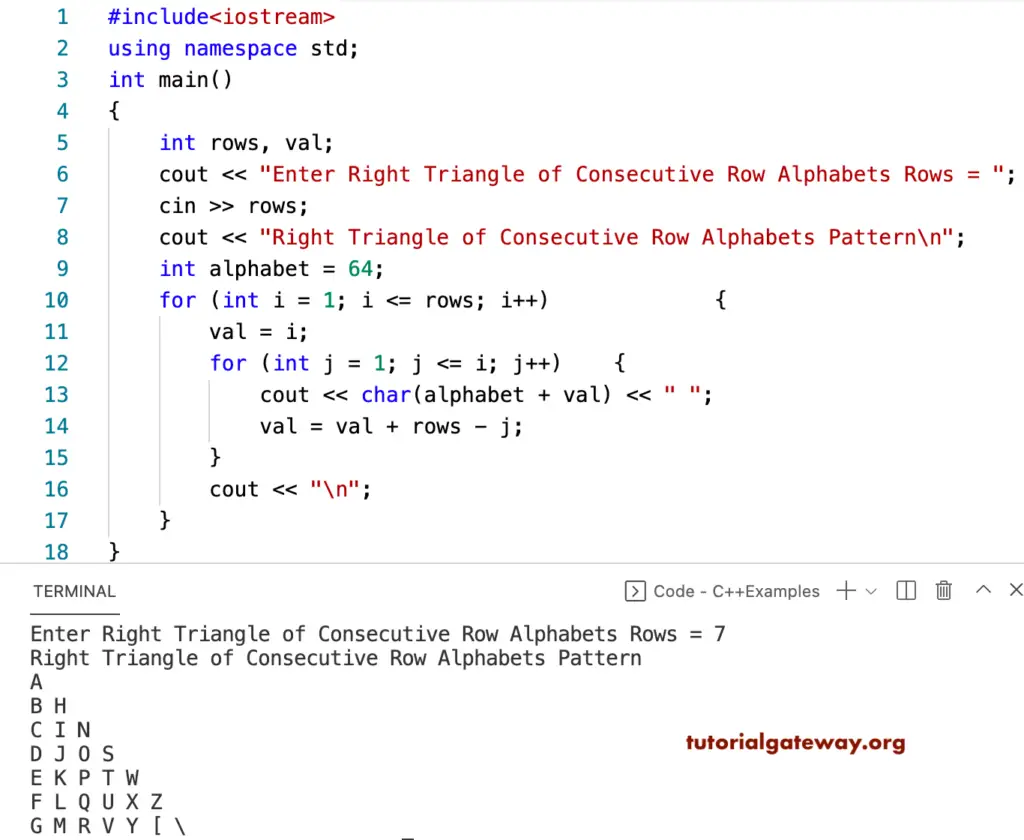
C++ program to print the right angled triangle of consecutive row alphabets pattern using a while loop.
#include<iostream> using namespace std; int main() { int rows, val, i, j, alphabet; cout << "Enter Right Triangle of Consecutive Row Alphabets Rows = "; cin >> rows; cout << "Right Triangle of Consecutive Row Alphabets Pattern\n"; alphabet = 64; i = 1; while (i <= rows) { val = i; j = 1; while (j <= i) { cout << char(alphabet + val) << " "; val = val + rows - j; j++; } cout << "\n"; i++; } }
Enter Right Triangle of Consecutive Row Alphabets Rows = 5
Right Triangle of Consecutive Row Alphabets Pattern
A
B F
C G J
D H K M
E I L N O
This C++ pattern example displays the right angled triangle of consecutive alphabets in each row using the do while loop.
#include<iostream> using namespace std; int main() { int rows, val, i, j, alphabet; cout << "Enter Right Triangle of Consecutive Row Alphabets Rows = "; cin >> rows; cout << "Right Triangle of Consecutive Row Alphabets Pattern\n"; alphabet = 64; i = 1; do { val = i; j = 1; do { cout << char(alphabet + val) << " "; val = val + rows - j; } while (++j <= i); cout << "\n"; } while (++i <= rows); }
Enter Right Triangle of Consecutive Row Alphabets Rows = 10
Right Triangle of Consecutive Row Alphabets Pattern
A
B K
C L T
D M U \
E N V ] c
F O W ^ d i
G P X _ e j n
H Q Y ` f k o r
I R Z a g l p s u
J S [ b h m q t v w