Write a C++ program to print right pascals triangle of multiplication numbers pattern using for loop.
#include<iostream> using namespace std; int main() { int rows, i, j; cout << "Enter Right Pascals Triangle Numeric Multiplication Rows = "; cin >> rows; cout << "The Right Pascals Triangle of Numbers Multiplication Pattern\n"; for (i = 1; i <= rows; i++) { for (j = 1; j <= i; j++) { cout << j * i << " "; } cout << "\n"; } for (i = rows - 1; i >= 1; i--) { for (j = 1; j <= i; j++) { cout << j * i << " "; } cout << "\n"; } }
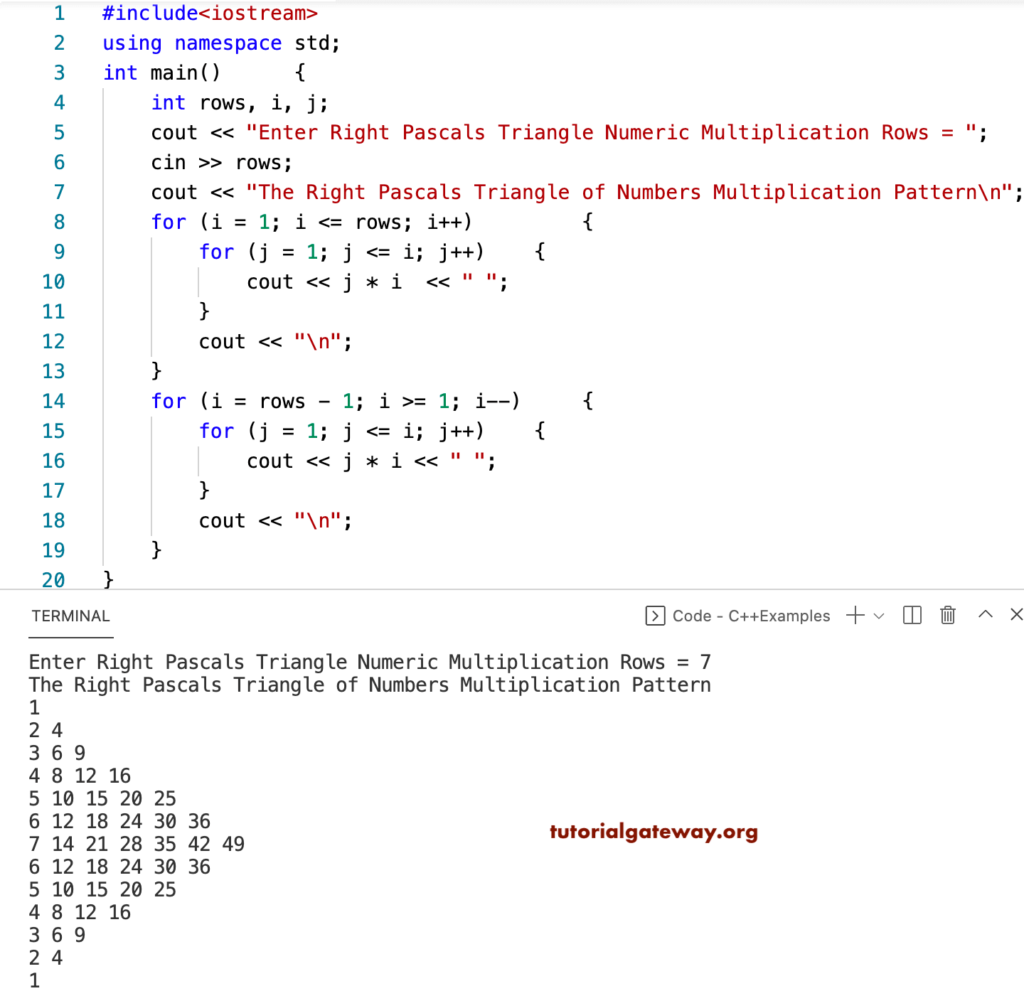
It is another way of writing the C++ program to print the right pascals triangle of multiplication numbers pattern.
#include<iostream> using namespace std; int main() { int rows, i, j, temp; cout << "Enter Right Pascals Triangle Numeric Multiplication Rows = "; cin >> rows; cout << "The Right Pascals Triangle of Numbers Multiplication Pattern\n"; temp = 1; for (i = 1; i <= rows / 2 + 1; i++, temp++) { for (j = 1; j <= i; j++) { cout << j * temp << " "; } cout << "\n"; } for (i = 1; i <= rows / 2; i++, temp++) { for (j = 1; j <= rows / 2 - i + 1; j++) { cout << j * temp << " "; } cout << "\n"; } }
Enter Right Pascals Triangle Numeric Multiplication Rows = 13
The Right Pascals Triangle of Numbers Multiplication Pattern
1
2 4
3 6 9
4 8 12 16
5 10 15 20 25
6 12 18 24 30 36
7 14 21 28 35 42 49
8 16 24 32 40 48
9 18 27 36 45
10 20 30 40
11 22 33
12 24
13
This C++ example displays the right pascals triangle pattern of multiplication numbers using the while loop.
#include<iostream> using namespace std; int main() { int rows, i, j, temp; cout << "Enter Right Pascals Numbers Multiplication Triangle Rows = "; cin >> rows; cout << "The Right Pascals Triangle of Numbers Multiplication Pattern\n"; i = temp = 1; while (i <= rows / 2 + 1) { j = 1; while (j <= i) { cout << j * temp << " "; j++; } cout << "\n"; temp++; i++; } i = 1; while (i <= rows / 2) { j = 1; while (j <= rows / 2 - i + 1) { cout << j * temp << " "; j++; } cout << "\n"; temp++; i++; } }
Enter Right Pascals Numbers Multiplication Triangle Rows = 15
The Right Pascals Triangle of Numbers Multiplication Pattern
1
2 4
3 6 9
4 8 12 16
5 10 15 20 25
6 12 18 24 30 36
7 14 21 28 35 42 49
8 16 24 32 40 48 56 64
9 18 27 36 45 54 63
10 20 30 40 50 60
11 22 33 44 55
12 24 36 48
13 26 39
14 28
15