Write a C++ program to print right pascals star triangle using for loop.
#include<iostream> using namespace std; int main() { int i, j, rows; cout << "Enter Right Pascals Star Triangle Rows = "; cin >> rows; cout << "Right Pascals Star Triangle Pattern\n"; for(i = 0; i < rows; i++) { for(j = 0; j <= i; j++) { cout << "* "; } cout << "\n"; } for(i = rows - 1; i >= 0; i--) { for(j = 0; j <= i - 1; j++) { cout << "* "; } cout << "\n"; } return 0; }
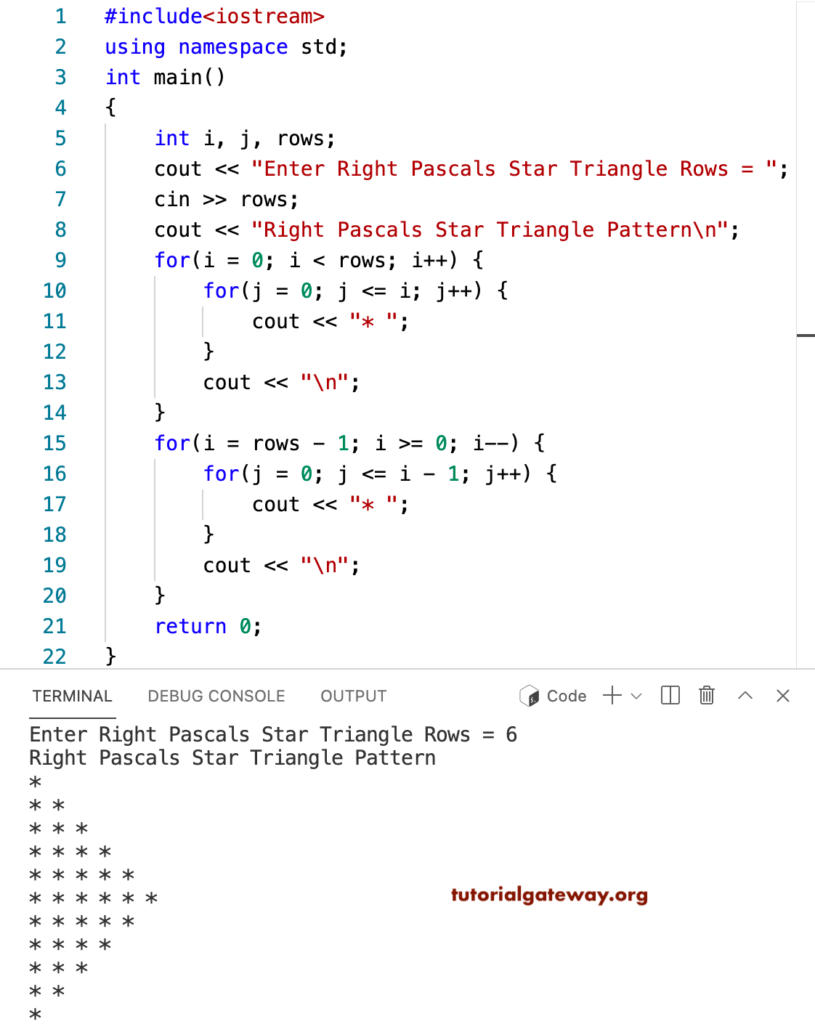
This C++ example prints the right pascals triangle pattern of a given character using a while loop.
#include<iostream> using namespace std; int main() { int i = 0, j, rows; char ch; cout << "Enter Right Pascals Star Triangle Rows = "; cin >> rows; cout << "Enter Symbol for Right Pascals Triangle = "; cin >> ch; cout << "Right Pascals Star Triangle Pattern\n"; while( i < rows) { j = 0; while( j <= i) { cout << ch << " "; j++; } cout << "\n"; i++; } i = rows - 1; while(i >= 0) { j = 0; while(j <= i - 1) { cout << ch << " "; j++; } cout << "\n"; i--; } return 0; }
Enter Right Pascals Star Triangle Rows = 12
Enter Symbol for Right Pascals Triangle = #
Right Pascals Star Triangle Pattern
#
# #
# # #
# # # #
# # # # #
# # # # # #
# # # # # # #
# # # # # # # #
# # # # # # # # #
# # # # # # # # # #
# # # # # # # # # # #
# # # # # # # # # # # #
# # # # # # # # # # #
# # # # # # # # # #
# # # # # # # # #
# # # # # # # #
# # # # # # #
# # # # # #
# # # # #
# # # #
# # #
# #
#