Write a C++ Program to Print Hollow Box Number Pattern using For Loop with an example. In this C++ example, we used the if statement inside the nested for loop to check for the box borders. If it is a box border, print 1; otherwise, print empty space.
#include<iostream> using namespace std; int main() { int i, j, rows, columns; cout << "\nPlease Enter the Number of Rows = "; cin >> rows; cout << "\nPlease Enter the Number of Columns = "; cin >> columns; cout << "\n---Hallow Box Number Pattern-----\n"; for(i = 1; i <= rows; i++) { for(j = 1; j <= columns; j++) { if(i == 1 || i == rows || j == 1 || j == columns) { cout << "1"; } else { cout << " "; } } cout << "\n"; } return 0; }
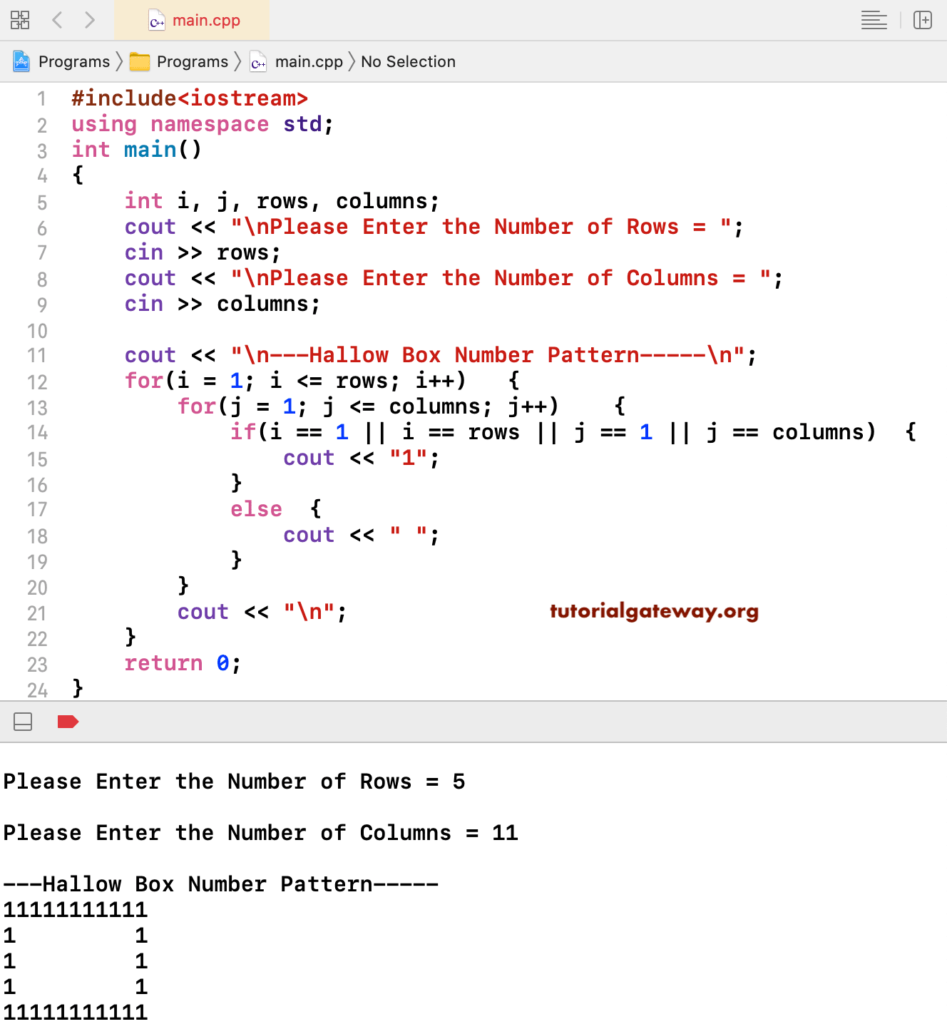
C++ Program to Print Hollow Box Pattern of Zeros
Here, we replaced 1 with 0.
#include<iostream> using namespace std; int main() { int i, j, rows, columns; cout << "\nPlease Enter the Number of Rows = "; cin >> rows; cout << "\nPlease Enter the Number of Columns = "; cin >> columns; cout << "\n---Hallow Box Number Pattern-----\n"; for(i = 1; i <= rows; i++) { for(j = 1; j <= columns; j++) { if(i == 1 || i == rows || j == 1 || j == columns) { cout << "0"; } else { cout << " "; } } cout << "\n"; } return 0; }
Please Enter the Number of Rows = 7
Please Enter the Number of Columns = 15
---Hallow Box Number Pattern-----
000000000000000
0 0
0 0
0 0
0 0
0 0
000000000000000
C++ Program to Print Hollow Box Number Pattern using While Loop
#include<iostream> using namespace std; int main() { int i, j, rows, columns; cout << "\nPlease Enter the Number of Rows = "; cin >> rows; cout << "\nPlease Enter the Number of Columns = "; cin >> columns; cout << "\n---Hollow Box Number Pattern-----\n"; i = 1; while(i <= rows) { j = 1; while(j <= columns) { if(i == 1 || i == rows || j == 1 || j == columns) { cout << "1"; } else { cout << " "; } j++; } cout << "\n"; i++; } return 0; }
Please Enter the Number of Rows = 7
Please Enter the Number of Columns = 16
---Hollow Box Number Pattern-----
1111111111111111
1 1
1 1
1 1
1 1
1 1
1111111111111111
In this C++ Hollow Box Number Pattern example, we allow the user to enter his/her own number to print in the borders.
#include<iostream> using namespace std; int main() { int i, j, rows, columns, num; cout << "\nPlease Enter the Number of Rows = "; cin >> rows; cout << "\nPlease Enter the Number of Columns = "; cin >> columns; cout << "\nPlease Enter Any Integer Value = "; cin >> num; cout << "\n---Hallow Box Number Pattern-----\n"; for(i = 1; i <= rows; i++) { for(j = 1; j <= columns; j++) { if(i == 1 || i == rows || j == 1 || j == columns) { cout << num; } else { cout << " "; } } cout << "\n"; } return 0; }
Please Enter the Number of Rows = 12
Please Enter the Number of Columns = 25
Please Enter Any Integer Value = 9
---Hallow Box Number Pattern-----
9999999999999999999999999
9 9
9 9
9 9
9 9
9 9
9 9
9 9
9 9
9 9
9 9
9999999999999999999999999