Write a C++ program to print first 10 odd natural numbers using for loop.
#include<iostream> using namespace std; int main() { cout << "The First 10 Odd Natural Numbers are\n"; for (int i = 1; i <= 10; i++) { cout << 2 * i - 1 << "\n"; } }
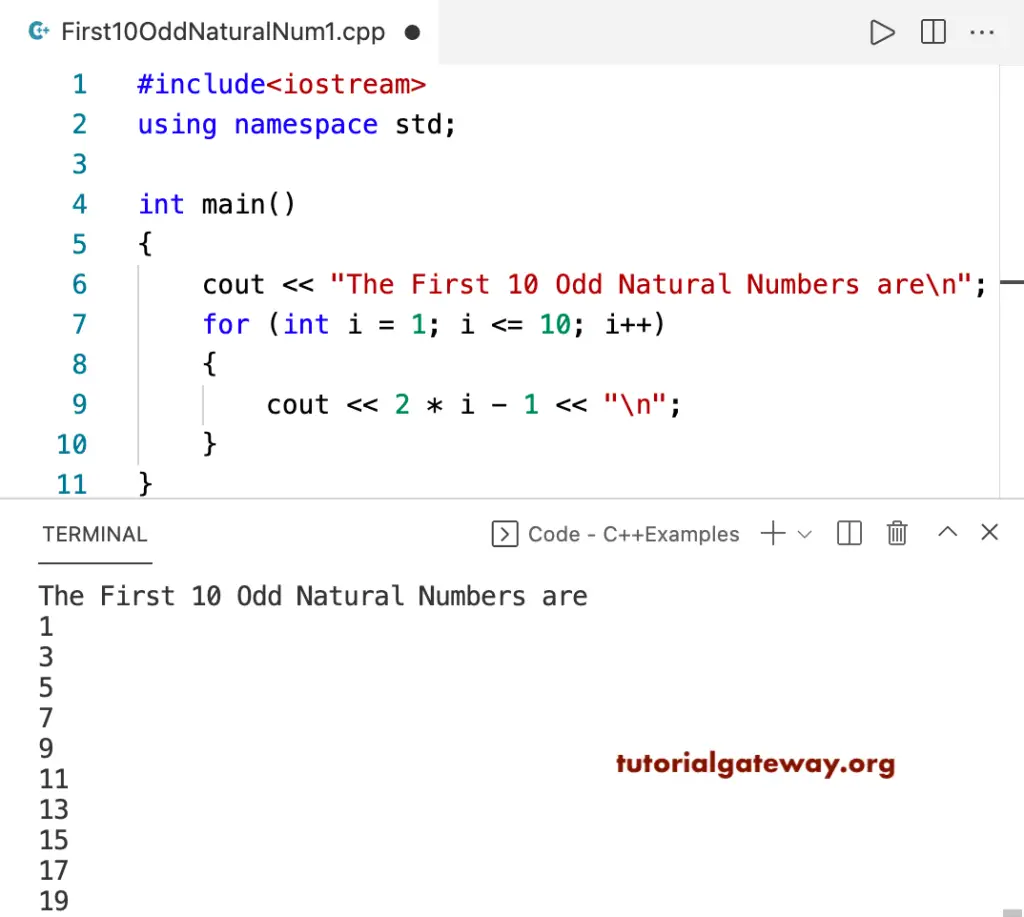
C++ program to print first 10 odd natural numbers using a while loop
#include<iostream> using namespace std; int main() { int i = 1; cout << "The First 10 Odd Natural Numbers are\n"; while (i <= 10) { cout << 2 * i - 1 << "\n"; i++; } }
The First 10 Odd Natural Numbers are
1
3
5
7
9
11
13
15
17
19
This C++ program uses the do while loop and displays the first 10 odd natural numbers.
#include<iostream> using namespace std; int main() { int i = 1; cout << "The First 10 Odd Natural Numbers are\n"; do { cout << 2 * i - 1 << "\n"; } while (++i <= 10); }
The First 10 Odd Natural Numbers are
1
3
5
7
9
11
13
15
17
19