Write a C++ program to find the largest number of the given three numbers. We used the Nested if statement in the below-shown program to find the largest of the three.
C++ Program to Find Largest of Three Numbers
The first if condition, if(x – y > 0 && x – z > 0) checks whether x is greater than y and z, if true, x is greater than y. We used a nested If else statement within the else blocks. if(y – z > 0) result is True, it prints y is greater than x and z. If the above conditions fail, z is greater than both x and y.
#include<iostream> using namespace std; int main() { int x, y, z; cout << "Please enter the Three Different Number = "; cin >> x >> y >> z; if(x - y > 0 && x - z > 0) { cout << x << " is Greater Than both " << y << " and " << z; } else { if(y - z > 0) { cout << y << " is Greater Than both " << x << " and " << z; } else { cout << z << " is Greater Than both " << x << " and " << y; } } return 0; }
Please enter the Three Different Number = 10 20 1
20 is Greater Than both 10 and 1
x= 44, y= 11, and z= 9
Please enter the Three Different Number = 44 11 9
44 is Greater Than both 11 and 9
x= 88, y= 77, and z= 122
Please enter the Three Different Number =
88
77
122
122 is Greater Than both 88 and 77
C++ Program to find Largest of Three Numbers using the Else If Statement
#include<iostream> using namespace std; int main() { int x, y, z; cout << "Please enter the Three Different Number = "; cin >> x >> y >> z; if (x > y && x > z) { cout << x << " is Greater Than both " << y << " and " << z; } else if (y > x && y > z) { cout << y << " is Greater Than both " << x << " and " << z; } else if (z > x && z > y) { cout << z << " is Greater Than both " << x << " and " << y; } else { cout << "Either any two variables are equal or all the three values are equal"; } return 0; }
Please enter the Three Different Number = 33 98 122
122 is Greater Than both 33 and 98
In this C++ example, we used a nested conditional operator (((x > y && x > z) ? x : (y > z) ? y : z).
#include<iostream> using namespace std; int main() { int x, y, z, largestOfThree; cout << "Please enter the Three Different Number = "; cin >> x >> y >> z; largestOfThree =((x > y && x > z) ? x : (y > z) ? y : z); cout << "\nLargest number among three is = " << largestOfThree; return 0; }
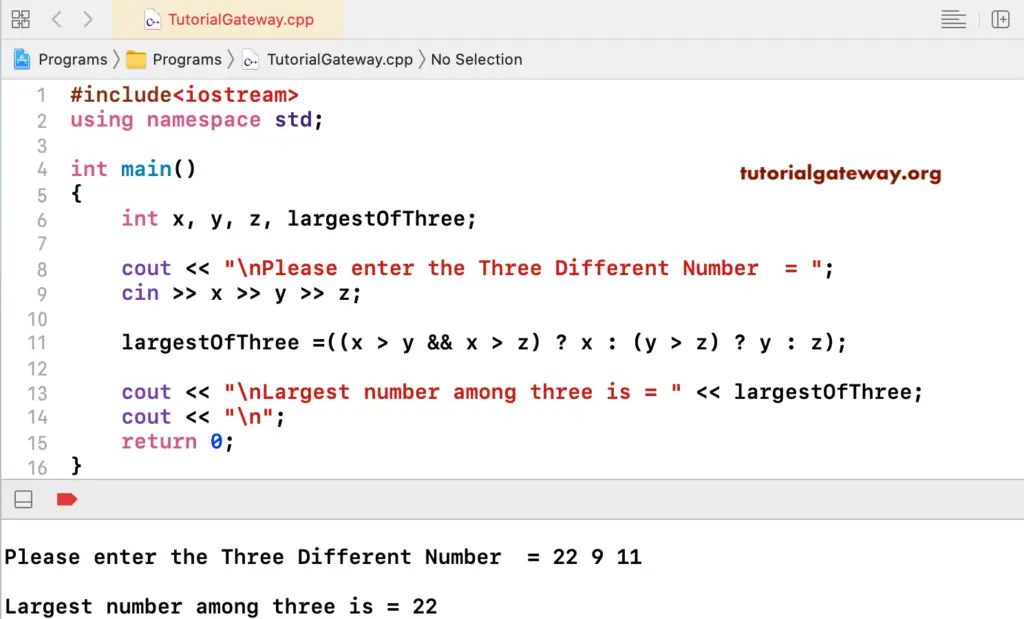