Write a C++ Program to find the Square of a Number with an example. This C++ code allows you to enter any number and finds the square of it.
#include<iostream> using namespace std; int main() { int number, square; cout << "\nPlease Enter Number to find Square of it = "; cin >> number; square = number * number; cout << "\nThe Square of the Given " << number << " = " << square; return 0; }
Please Enter Number to find Square of it = 5
The Square of the Given 5 = 25
C++ Program to Calculate Square of a Number using Functions
#include<iostream> using namespace std; int calculateSquare(int number) { return number * number; } int main() { int number; cout << "\nPlease Enter Number to find Square of it = "; cin >> number; int square = calculateSquare(number); cout << "\nThe Square of the Given " << number << " = " << square; return 0; }
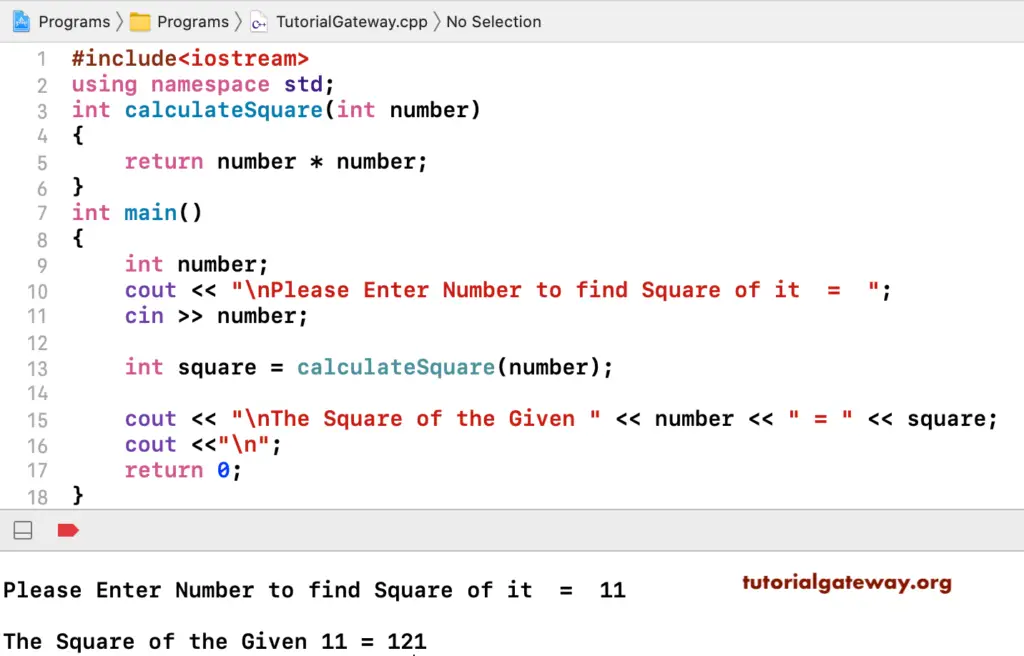