Regular Expressions help to describe complex patterns in texts. These C# regular Expressions are commonly known as RegEx in short. The first thing we need to understand is in the C# Regex expression; everything is essentially a character.
The described C# Regex patterns could be used to search, extract, replace, and modify text data. C# supports regular expressions by providing classes in System.Text.RegularExpressions namespace.
C# Regular Expressions are nothing but patterns. After defining patterns, we can validate them by searching, extracting, replacing, and modifying them.
In general, every structured data have some specific pattern. Let us say, for example,
www.tutorialgateway.org
Any URL has some specific pattern, like it starts with www and ends with org and, in between, connected with dots.
contact@tutorialgateway.org
Likewise, email id also has a specific pattern like
It starts with some alphanumeric characters, and then there will be a ‘@’ symbol after that. Again there may be alphanumeric characters followed by a dot, which is again followed by ‘com’, or ‘org’, etc.
7/12/2018(mm/dd/yyyy)
Date format has a certain pattern like mm-dd-yy format or mm-dd-yyyy format. The C# Regular expressions help us to define such patterns.
C# Regular expressions look something like the one below. The C# Regex for simple email validation is.
^[a-zA-Z0-9]{1,10}@[a-zA-Z0-9]{1,10}.(com|org|in)$
Let us see the basic information to write c# regular expressions.
B for Brackets, C for Carrot, D for Dollar
B | There are three types of brackets used in C# regular expression “[“ and curly “{“ braces. Square brackets specify the character which has to be matched, while curly brackets specify how many characters. “(“ is for grouping. |
C | Carrot “^” is the start of a regular expression |
D | Dollar “$” the end of the regular expression |
C# Regex or Regular Expressions Examples
The following examples show some of the simple C# regular expression patterns.
Enter the character that exists between a-g.
[a-g] –
Enter a character that exists between a-g with a length of 3.
Regex: ^[a-g]{3}$ –
Enter a character between a-g with a max of three characters and a minimum of one character.
Regex: ^[a-g]{1,3}$
Validating data with 7 digits fix numerical format like 8743524, 6864351, etc.
Regex: ^[0-9]{7}$
How do we validate numeric data with three as the minimum and six as the maximum length?
^[0-9]{3,7}$
How to validate invoice numbers with formats like KTG5240, the first three are alphabetical characters, and the remaining is the length of 6 numbers.
^[a-z]{3)[0-9]{6}$
Let us see a simple C# Regex for validating the URL.
Regex: ^www.[A-Za-z0-9]{1,10}.(com|org|in)$
Using verbatim literal instead of regular strings while writing regular expressions in C# is always recommended.
Verbatim literals start with the special prefix (@) that helps not to interpret backslashes and meta characters in the string.
For instance, instead of writing \\m\\n, we can write it as @” \n\m”, which makes the string readable.
C# Regex String Matching
The C# Regex is a class in the namespace System.Text.RegularExpressions envelop the interfaces to the regular expressions engine, thus allowing us to perform matches and extract data from text using regular expressions.
In this C# language, there is a static method, Regex.Match to test whether the Regex matches a string or not. The enum Regexoptions is an optional setting to the method Regex.Match. The Regex.Match returns a Match object which holds the information of where the match was found if there is a match.
The C# Regex string match syntax is
Match match = Regex.Match(InputStr, Pattern, RegexOptions)
Let us see an example to demonstrate the C# Regular expressions. In this example, we are demonstrating the regex pattern for “august 25”.
using System; using System.Text.RegularExpressions; class program { public static void Main(string[] args) { string pattern = @"([a-zA-Z]+) (\d+)"; string input = "special occasion on august 25"; Match match = Regex.Match(input, pattern); if(match.Success) { Console.WriteLine(match.Value); } Console.ReadKey(); } }
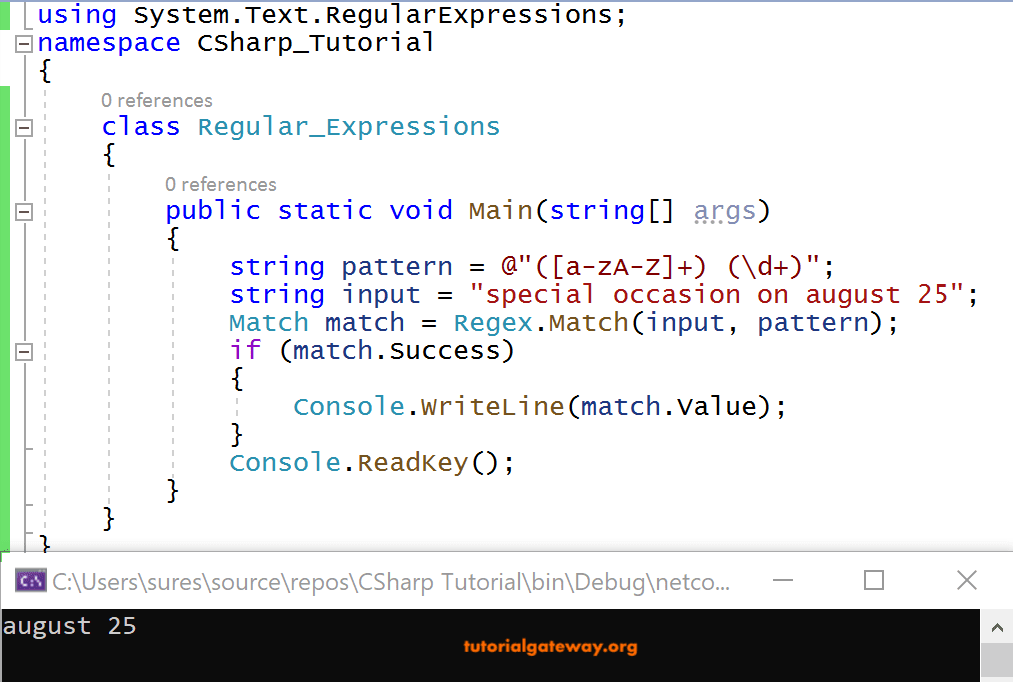
ANALYSIS
We have taken a string variable pattern to store the regex pattern. Next, the input is another string variable to store the text we want to search for the pattern match. And the match is a variable of type Match to store the result of the static method regex.Match returns. If the match succeeds, the match value is printed onto the console.
C# RegEx or Regular Expressions patterns
The following table shows the list of common RegEx patterns and their descriptions.
abc… | Letters |
123… | Digits |
\d | Any Digit |
\D | Any Non-digit character |
. | Any Character |
\. | Period |
[abc] | Only a, b, or c |
[^abc] | Not a, b, nor c |
[a-z] | Characters a to z |
[0-9] | Numbers 0 to 9 |
\w | Any Alphanumeric character |
\W | Any Non-alphanumeric character |
{m} | m Repetitions |
{m,n} | m to n Repetitions |
* | Zero or more repetitions |
+ | One or more repetitions |
? | Optional character |
\s | Any Whitespace |
\S | Any Non-whitespace character |
^…$ | Starts and ends |
(…) | Capture Group |
(a(bc)) | Capture Sub-group |
(.*) | Capture all |
(abc|def) | Matches abc or def |
Let us see another code demonstrating the C# Regex regular expression pattern for validating simple email id contact@tutorialgateway.org
using System; using System.Text.RegularExpressions; class program { public static void Main(string[] args) { string pattern = "[A-Za-z0-9]{1,20}@[a-zA-Z]{1,20}.(com|org)$"; string input = "Website is www.tutorialgateway.org, contact us at contact@tutorialgateway.org"; Match match = Regex.Match(input, pattern); if(match.Success) { Console.WriteLine(match.Value); } Console.ReadKey(); } }
OUTPUT
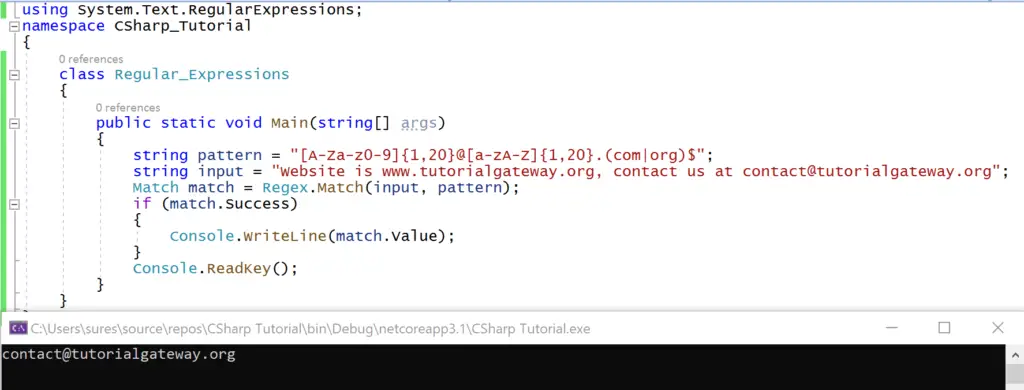
ANALYSIS
As you have seen in the above example code, the string variable pattern is to store the regex pattern. Variable input is to store the text we give.
Both pattern and input are passed as arguments to the Regex.Match method returns the match value if the match is successful.